Welcome to the third section dedicated to alfresco tests. This guide will allow you to test the web scripts created within an alfresco distribution without having to do any integration tests. The test can be performed directly as a unitary test without therefore having to start distributing alfresco.
To do this we will use alfresco-tests, a library that contains the alfresco services mocked and rebuilt just for testing. On this page you will find the details of the library.
Project scheme
In the photo, we see the web script inside alfresco that we are going to test inside our SDK 4.1.
First, let’s give a brief mention of the structure of the project in the SDK you see in the following photo:
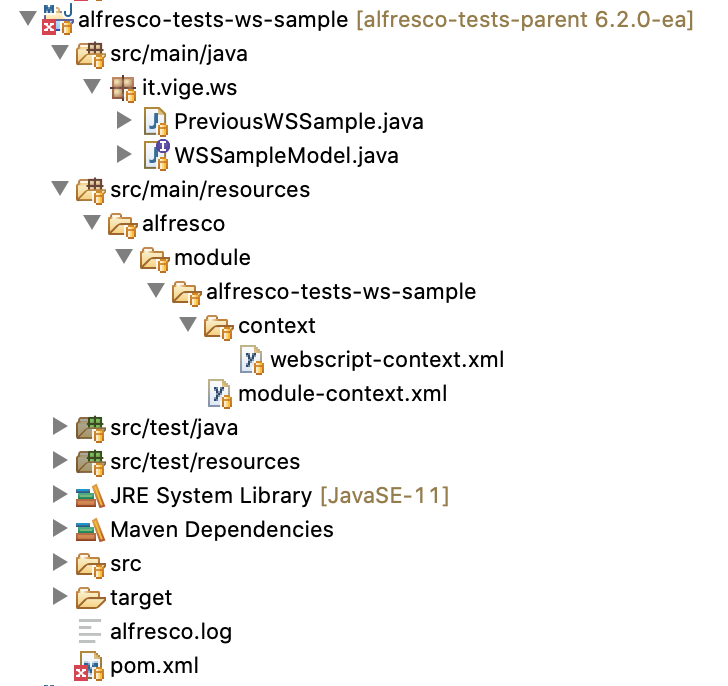
The following elements are present:
pom.xml – The descriptor of the SDK project
webscript-context.xml – collects the alfresco components used by the web script
module-context.xml – The main descriptor of the alfresco module that calls properties and components
PreviousWSSample.java – A sample declarative web script, written in Java
WSSampleModel.java – An interface with the namespaces and the used qnames
For details you can see the project in the github repository.
To start we write the spring descriptor test-module-context.xml and place it in the src / test / resources folder of our project
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<import resource="classpath:test-ws-context.xml" />
<import
resource="classpath:alfresco/module/${project.artifactId}/context/webscript-context.xml" />
</beans>
test-ws-context.xml is the default descriptor in the alfresco-tests library. It let to load all web script default components used to start the web script context.
webscript-context.xml is the descriptor already present in our project of the web script and all components used in the web script
JUnit Test
Now we will write the junit test to put in the src/test/java path:
import org.alfresco.mock.test.ws.AbstractWSForm;
import org.junit.Test;
import org.junit.runner.RunWith;
import com.tradeshift.test.remote.Remote;
import com.tradeshift.test.remote.RemoteTestRunner;
import org.springframework.test.context.ContextConfiguration;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
...
@RunWith(RemoteTestRunner.class)
@Remote(runnerClass = SpringJUnit4ClassRunner.class)
@ContextConfiguration("classpath:test-module-context.xml")
public class PreviousWSSampleTest extends AbstractWSForm {
@Test
public void execute() throws ParseException, IOException {
}
}
AbstractWSForm class allows you to communicate with the alfresco-tests library and initialize the test environment.
The @RunWith and @Remote annotations are used to initialize the junit test and through the @ContextConfiguration annotation load the spring context declared in the test-module-context.xml file descriptor.
We can add the init method to add our documents and content nodes. Here a sample:
import org.alfresco.service.cmr.repository.NodeRef;
import org.alfresco.service.namespace.NamespaceService;
import org.junit.Before;
import it.vige.ws.WSSampleModel;
...
private final static String CARTELLA_WSSAMPLE = "WSSAMPLE-20157726";
private NodeRef repository;
...
@Before
public void init() {
super.init();
NamespaceService namespaceService = serviceRegistry.getNamespaceService();
namespaceService.registerNamespace("mccpb",
WSSampleModel.PBPDV_NAMESPACE);
// Creating initial folders and sites
NodeRef site = insertFolder(sites, "digital-conservation");
insertFolder(site, "documentLibrary");
NodeRef bankSite = insertFolder(sites, "bank-site");
NodeRef bankSiteDL = insertFolder(bankSite, "documentLibrary");
repository = insertFolder(bankSiteDL, "repository");
insertFolder(repository, CARTELLA_WSSAMPLE);
}
Thanks to the alfresco-tests library, ready-made methods are provided such as insertFolder used to create new folders. Also the serviceRegistry variable is inherited by the AlfrescoWSForm class and it let to communicate with the alfresco repository.
Also we import the webscript module and declare it
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.extensions.webscripts.AbstractWebScript;
...
@Autowired
private PreviousWSSample previousWSSample;
@Override
protected AbstractWebScript getAbstractWebScript() {
return previousWSSample;
}
Now we are ready to implement the main execute() method
import java.util.HashMap;
import java.util.List;
import org.alfresco.service.cmr.repository.NodeService;
import org.alfresco.service.cmr.search.SearchService;
import org.springframework.extensions.webscripts.WebScriptRequest;
import org.alfresco.mock.test.ws.MockWebScriptRequest;
import org.alfresco.mock.test.ws.MockWebScriptResponse;
import org.alfresco.service.namespace.QName;
import org.junit.Assert;
...
SearchService searchService = serviceRegistry.getSearchService();
NodeService nodeService = serviceRegistry.getNodeService();
Map<String, Serializable> fields = new HashMap<String, Serializable>();
{
fields.put("date_modified", dataModifica);
fields.put("date_ws_end", dataModifica);
fields.put("codicews", CARTELLA_WSSAMPLE);
fields.put("date_ws_start", "1970-01-01");
}
WebScriptRequest webScriptRequest = new MockWebScriptRequest("json", null,
previousWSSample, fields, serviceRegistry);
previousWSSample.execute(webScriptRequest, new MockWebScriptResponse());
// Verify
List<NodeRef> nodeRefs = searchService
.query(StoreRef.STORE_REF_WORKSPACE_SPACESSTORE,
SearchService.LANGUAGE_XPATH, CARTELLA_WSSAMPLE)
.getNodeRefs();
NodeRef result = nodeRefs.get(0);
Set<QName> aspects = nodeService.getAspects(result);
Assert.assertEquals("One aspect for the folder", 1, aspects.size());
QName aspect = aspects.iterator().next();
Assert.assertEquals("Added an aspect to the WS Sample folder",
WSSampleModel.ASPECT_WSSAMPLEFOLDER, aspect);
Date date = (Date) nodeService.getProperty(result,
WSSampleModel.PROP_UPDATE_PROPERTY);
Assert.assertEquals("Added the date property", dataModifica,
dateFormat.format(date));
Mainly to run the webscript it is enough to do two things.
First instantiate the WebScriptRequest request via the mock implementation MockWebScriptRequest by also entering the parameters of the web service.
Second instantiate a response with a simple new MockWebScriptResponse ()
Install the Test Library
Now to make the test work we need to integrate the alfresco-tests library into the pom.xml. Simply in the dependencies we add:
<dependency>
<groupId>it.vige</groupId>
<artifactId>alfresco-tests</artifactId>
<version>6.2.0-ea.6</version>
<scope>test</scope>
</dependency>
The version number is dependent on the alfresco version. In this case, we are using alfresco 6.2.0-ea.
All is done. Now you can start the test